JsWebrtc文档
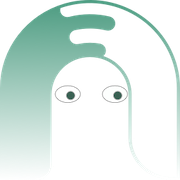
JSWebrtc – 支持SRS的 Webrtc 播放器
JSWebrtc 对浏览器的 Webrtc 做了简单的封装,支持 SRS 的 RTC 流的播放.
1interface RTCPeerConnectionEventMap {
2 "connectionstatechange": Event;
3 "datachannel": RTCDataChannelEvent;
4 "icecandidate": RTCPeerConnectionIceEvent;
5 "icecandidateerror": Event;
6 "iceconnectionstatechange": Event;
7 "icegatheringstatechange": Event;
8 "negotiationneeded": Event;
9 "signalingstatechange": Event;
10 "track": RTCTrackEvent;
11}
1/** A WebRTC connection between the local computer and a remote peer. It provides methods to connect to a remote peer, maintain and monitor the connection, and close the connection once it's no longer needed. */
2interface RTCPeerConnection extends EventTarget {
3 readonly canTrickleIceCandidates: boolean | null;
4 readonly connectionState: RTCPeerConnectionState;
5 readonly currentLocalDescription: RTCSessionDescription | null;
6 readonly currentRemoteDescription: RTCSessionDescription | null;
7 readonly iceConnectionState: RTCIceConnectionState;
8 readonly iceGatheringState: RTCIceGatheringState;
9 readonly localDescription: RTCSessionDescription | null;
10 onconnectionstatechange: ((this: RTCPeerConnection, ev: Event) => any) | null;
11 ondatachannel: ((this: RTCPeerConnection, ev: RTCDataChannelEvent) => any) | null;
12 onicecandidate: ((this: RTCPeerConnection, ev: RTCPeerConnectionIceEvent) => any) | null;
13 onicecandidateerror: ((this: RTCPeerConnection, ev: Event) => any) | null;
14 oniceconnectionstatechange: ((this: RTCPeerConnection, ev: Event) => any) | null;
15 onicegatheringstatechange: ((this: RTCPeerConnection, ev: Event) => any) | null;
16 onnegotiationneeded: ((this: RTCPeerConnection, ev: Event) => any) | null;
17 onsignalingstatechange: ((this: RTCPeerConnection, ev: Event) => any) | null;
18 ontrack: ((this: RTCPeerConnection, ev: RTCTrackEvent) => any) | null;
19 readonly pendingLocalDescription: RTCSessionDescription | null;
20 readonly pendingRemoteDescription: RTCSessionDescription | null;
21 readonly remoteDescription: RTCSessionDescription | null;
22 readonly signalingState: RTCSignalingState;
23 addIceCandidate(candidate?: RTCIceCandidateInit): Promise<void>;
24 /** @deprecated */
25 addIceCandidate(candidate: RTCIceCandidateInit, successCallback: VoidFunction, failureCallback: RTCPeerConnectionErrorCallback): Promise<void>;
26 addTrack(track: MediaStreamTrack, ...streams: MediaStream[]): RTCRtpSender;
27 addTransceiver(trackOrKind: MediaStreamTrack | string, init?: RTCRtpTransceiverInit): RTCRtpTransceiver;
28 close(): void;
29 createAnswer(options?: RTCAnswerOptions): Promise<RTCSessionDescriptionInit>;
30 /** @deprecated */
31 createAnswer(successCallback: RTCSessionDescriptionCallback, failureCallback: RTCPeerConnectionErrorCallback): Promise<void>;
32 createDataChannel(label: string, dataChannelDict?: RTCDataChannelInit): RTCDataChannel;
33 createOffer(options?: RTCOfferOptions): Promise<RTCSessionDescriptionInit>;
34 /** @deprecated */
35 createOffer(successCallback: RTCSessionDescriptionCallback, failureCallback: RTCPeerConnectionErrorCallback, options?: RTCOfferOptions): Promise<void>;
36 getConfiguration(): RTCConfiguration;
37 getReceivers(): RTCRtpReceiver[];
38 getSenders(): RTCRtpSender[];
39 getStats(selector?: MediaStreamTrack | null): Promise<RTCStatsReport>;
40 getTransceivers(): RTCRtpTransceiver[];
41 removeTrack(sender: RTCRtpSender): void;
42 restartIce(): void;
43 setConfiguration(configuration?: RTCConfiguration): void;
44 setLocalDescription(description?: RTCLocalSessionDescriptionInit): Promise<void>;
45 /** @deprecated */
46 setLocalDescription(description: RTCLocalSessionDescriptionInit, successCallback: VoidFunction, failureCallback: RTCPeerConnectionErrorCallback): Promise<void>;
47 setRemoteDescription(description: RTCSessionDescriptionInit): Promise<void>;
48 /** @deprecated */
49 setRemoteDescription(description: RTCSessionDescriptionInit, successCallback: VoidFunction, failureCallback: RTCPeerConnectionErrorCallback): Promise<void>;
50 addEventListener<K extends keyof RTCPeerConnectionEventMap>(type: K, listener: (this: RTCPeerConnection, ev: RTCPeerConnectionEventMap[K]) => any, options?: boolean | AddEventListenerOptions): void;
51 addEventListener(type: string, listener: EventListenerOrEventListenerObject, options?: boolean | AddEventListenerOptions): void;
52 removeEventListener<K extends keyof RTCPeerConnectionEventMap>(type: K, listener: (this: RTCPeerConnection, ev: RTCPeerConnectionEventMap[K]) => any, options?: boolean | EventListenerOptions): void;
53 removeEventListener(type: string, listener: EventListenerOrEventListenerObject, options?: boolean | EventListenerOptions): void;
54}
1declare var RTCPeerConnection: {
2 prototype: RTCPeerConnection;
3 new(configuration?: RTCConfiguration): RTCPeerConnection;
4 generateCertificate(keygenAlgorithm: AlgorithmIdentifier): Promise<RTCCertificate>;
5};
1interface RTCPeerConnectionIceErrorEvent extends Event {
2 readonly address: string | null;
3 readonly errorCode: number;
4 readonly errorText: string;
5 readonly port: number | null;
6 readonly url: string;
7}
1declare var RTCPeerConnectionIceErrorEvent: {
2 prototype: RTCPeerConnectionIceErrorEvent;
3 new(type: string, eventInitDict: RTCPeerConnectionIceErrorEventInit): RTCPeerConnectionIceErrorEvent;
4};
1/** Events that occurs in relation to ICE candidates with the target, usually an RTCPeerConnection. Only one event is of this type: icecandidate. */
2interface RTCPeerConnectionIceEvent extends Event {
3 readonly candidate: RTCIceCandidate | null;
4}
1declare var RTCPeerConnectionIceEvent: {
2 prototype: RTCPeerConnectionIceEvent;
3 new(type: string, eventInitDict?: RTCPeerConnectionIceEventInit): RTCPeerConnectionIceEvent;
4};
1/** This WebRTC API interface manages the reception and decoding of data for a MediaStreamTrack on an RTCPeerConnection. */
2interface RTCRtpReceiver {
3 readonly track: MediaStreamTrack;
4 readonly transport: RTCDtlsTransport | null;
5 getContributingSources(): RTCRtpContributingSource[];
6 getParameters(): RTCRtpReceiveParameters;
7 getStats(): Promise<RTCStatsReport>;
8 getSynchronizationSources(): RTCRtpSynchronizationSource[];
9}
1declare var RTCRtpReceiver: {
2 prototype: RTCRtpReceiver;
3 new(): RTCRtpReceiver;
4 getCapabilities(kind: string): RTCRtpCapabilities | null;
5};
1/** Provides the ability to control and obtain details about how a particular MediaStreamTrack is encoded and sent to a remote peer. */
2interface RTCRtpSender {
3 readonly dtmf: RTCDTMFSender | null;
4 readonly track: MediaStreamTrack | null;
5 readonly transport: RTCDtlsTransport | null;
6 getParameters(): RTCRtpSendParameters;
7 getStats(): Promise<RTCStatsReport>;
8 replaceTrack(withTrack: MediaStreamTrack | null): Promise<void>;
9 setParameters(parameters: RTCRtpSendParameters): Promise<void>;
10 setStreams(...streams: MediaStream[]): void;
11}
1declare var RTCRtpSender: {
2 prototype: RTCRtpSender;
3 new(): RTCRtpSender;
4 getCapabilities(kind: string): RTCRtpCapabilities | null;
5};
1interface RTCRtpTransceiver {
2 readonly currentDirection: RTCRtpTransceiverDirection | null;
3 direction: RTCRtpTransceiverDirection;
4 readonly mid: string | null;
5 readonly receiver: RTCRtpReceiver;
6 readonly sender: RTCRtpSender;
7 setCodecPreferences(codecs: RTCRtpCodecCapability[]): void;
8 stop(): void;
9}
1declare var RTCRtpTransceiver: {
2 prototype: RTCRtpTransceiver;
3 new(): RTCRtpTransceiver;
4};
1/** One end of a connection—or potential connection—and how it's configured. Each RTCSessionDescription consists of a description type indicating which part of the offer/answer negotiation process it describes and of the SDP descriptor of the session. */
2interface RTCSessionDescription {
3 readonly sdp: string;
4 readonly type: RTCSdpType;
5 toJSON(): any;
6}
1declare var RTCSessionDescription: {
2 prototype: RTCSessionDescription;
3 new(descriptionInitDict: RTCSessionDescriptionInit): RTCSessionDescription;
4};
1interface RTCStatsReport {
2 forEach(callbackfn: (value: any, key: string, parent: RTCStatsReport) => void, thisArg?: any): void;
3}
1declare var RTCStatsReport: {
2 prototype: RTCStatsReport;
3 new(): RTCStatsReport;
4};
1interface RTCTrackEvent extends Event {
2 readonly receiver: RTCRtpReceiver;
3 readonly streams: ReadonlyArray<MediaStream>;
4 readonly track: MediaStreamTrack;
5 readonly transceiver: RTCRtpTransceiver;
6}
1declare var RTCTrackEvent: {
2 prototype: RTCTrackEvent;
3 new(type: string, eventInitDict: RTCTrackEventInit): RTCTrackEvent;
4};
1interface RTCAnswerOptions extends RTCOfferAnswerOptions {
2}
3
4interface RTCCertificateExpiration {
5 expires?: number;
6}
7
8interface RTCConfiguration {
9 bundlePolicy?: RTCBundlePolicy;
10 certificates?: RTCCertificate[];
11 iceCandidatePoolSize?: number;
12 iceServers?: RTCIceServer[];
13 iceTransportPolicy?: RTCIceTransportPolicy;
14 rtcpMuxPolicy?: RTCRtcpMuxPolicy;
15}
16
17interface RTCDTMFToneChangeEventInit extends EventInit {
18 tone?: string;
19}
20
21interface RTCDataChannelEventInit extends EventInit {
22 channel: RTCDataChannel;
23}
24
25interface RTCDataChannelInit {
26 id?: number;
27 maxPacketLifeTime?: number;
28 maxRetransmits?: number;
29 negotiated?: boolean;
30 ordered?: boolean;
31 protocol?: string;
32}
33
34interface RTCDtlsFingerprint {
35 algorithm?: string;
36 value?: string;
37}
38
39interface RTCIceCandidateInit {
40 candidate?: string;
41 sdpMLineIndex?: number | null;
42 sdpMid?: string | null;
43 usernameFragment?: string | null;
44}
45
46interface RTCIceCandidatePairStats extends RTCStats {
47 availableIncomingBitrate?: number;
48 availableOutgoingBitrate?: number;
49 bytesReceived?: number;
50 bytesSent?: number;
51 currentRoundTripTime?: number;
52 localCandidateId: string;
53 nominated?: boolean;
54 remoteCandidateId: string;
55 requestsReceived?: number;
56 requestsSent?: number;
57 responsesReceived?: number;
58 responsesSent?: number;
59 state: RTCStatsIceCandidatePairState;
60 totalRoundTripTime?: number;
61 transportId: string;
62}
63
64interface RTCIceServer {
65 credential?: string;
66 credentialType?: RTCIceCredentialType;
67 urls: string | string[];
68 username?: string;
69}
70
71interface RTCInboundRtpStreamStats extends RTCReceivedRtpStreamStats {
72 firCount?: number;
73 framesDecoded?: number;
74 nackCount?: number;
75 pliCount?: number;
76 qpSum?: number;
77 remoteId?: string;
78}
79
80interface RTCLocalSessionDescriptionInit {
81 sdp?: string;
82 type?: RTCSdpType;
83}
84
85interface RTCOfferAnswerOptions {
86}
87
88interface RTCOfferOptions extends RTCOfferAnswerOptions {
89 iceRestart?: boolean;
90 offerToReceiveAudio?: boolean;
91 offerToReceiveVideo?: boolean;
92}
93
94interface RTCOutboundRtpStreamStats extends RTCSentRtpStreamStats {
95 firCount?: number;
96 framesEncoded?: number;
97 nackCount?: number;
98 pliCount?: number;
99 qpSum?: number;
100 remoteId?: string;
101}
102
103interface RTCPeerConnectionIceErrorEventInit extends EventInit {
104 address?: string | null;
105 errorCode: number;
106 errorText?: string;
107 port?: number | null;
108 url?: string;
109}
110
111interface RTCPeerConnectionIceEventInit extends EventInit {
112 candidate?: RTCIceCandidate | null;
113 url?: string | null;
114}
115
116interface RTCReceivedRtpStreamStats extends RTCRtpStreamStats {
117 jitter?: number;
118 packetsDiscarded?: number;
119 packetsLost?: number;
120 packetsReceived?: number;
121}
122
123interface RTCRtcpParameters {
124 cname?: string;
125 reducedSize?: boolean;
126}
127
128interface RTCRtpCapabilities {
129 codecs: RTCRtpCodecCapability[];
130 headerExtensions: RTCRtpHeaderExtensionCapability[];
131}
132
133interface RTCRtpCodecCapability {
134 channels?: number;
135 clockRate: number;
136 mimeType: string;
137 sdpFmtpLine?: string;
138}
139
140interface RTCRtpCodecParameters {
141 channels?: number;
142 clockRate: number;
143 mimeType: string;
144 payloadType: number;
145 sdpFmtpLine?: string;
146}
147
148interface RTCRtpCodingParameters {
149 rid?: string;
150}
151
152interface RTCRtpContributingSource {
153 audioLevel?: number;
154 rtpTimestamp: number;
155 source: number;
156 timestamp: DOMHighResTimeStamp;
157}
158
159interface RTCRtpEncodingParameters extends RTCRtpCodingParameters {
160 active?: boolean;
161 maxBitrate?: number;
162 priority?: RTCPriorityType;
163 scaleResolutionDownBy?: number;
164}
165
166interface RTCRtpHeaderExtensionCapability {
167 uri?: string;
168}
169
170interface RTCRtpHeaderExtensionParameters {
171 encrypted?: boolean;
172 id: number;
173 uri: string;
174}
175
176interface RTCRtpParameters {
177 codecs: RTCRtpCodecParameters[];
178 headerExtensions: RTCRtpHeaderExtensionParameters[];
179 rtcp: RTCRtcpParameters;
180}
181
182interface RTCRtpReceiveParameters extends RTCRtpParameters {
183}
184
185interface RTCRtpSendParameters extends RTCRtpParameters {
186 degradationPreference?: RTCDegradationPreference;
187 encodings: RTCRtpEncodingParameters[];
188 transactionId: string;
189}
190
191interface RTCRtpStreamStats extends RTCStats {
192 codecId?: string;
193 kind: string;
194 ssrc: number;
195 transportId?: string;
196}
197
198interface RTCRtpSynchronizationSource extends RTCRtpContributingSource {
199}
200
201interface RTCRtpTransceiverInit {
202 direction?: RTCRtpTransceiverDirection;
203 sendEncodings?: RTCRtpEncodingParameters[];
204 streams?: MediaStream[];
205}
206
207interface RTCSentRtpStreamStats extends RTCRtpStreamStats {
208 bytesSent?: number;
209 packetsSent?: number;
210}
211
212interface RTCSessionDescriptionInit {
213 sdp?: string;
214 type: RTCSdpType;
215}
216
217interface RTCStats {
218 id: string;
219 timestamp: DOMHighResTimeStamp;
220 type: RTCStatsType;
221}
222
223interface RTCTrackEventInit extends EventInit {
224 receiver: RTCRtpReceiver;
225 streams?: MediaStream[];
226 track: MediaStreamTrack;
227 transceiver: RTCRtpTransceiver;
228}
229
230interface RTCTransportStats extends RTCStats {
231 bytesReceived?: number;
232 bytesSent?: number;
233 dtlsCipher?: string;
234 dtlsState: RTCDtlsTransportState;
235 localCertificateId?: string;
236 remoteCertificateId?: string;
237 rtcpTransportStatsId?: string;
238 selectedCandidatePairId?: string;
239 srtpCipher?: string;
240 tlsVersion?: string;
241}
242
243
244type RTCBundlePolicy = "balanced" | "max-bundle" | "max-compat";
245type RTCDataChannelState = "closed" | "closing" | "connecting" | "open";
246type RTCDegradationPreference = "balanced" | "maintain-framerate" | "maintain-resolution";
247type RTCDtlsTransportState = "closed" | "connected" | "connecting" | "failed" | "new";
248type RTCIceCandidateType = "host" | "prflx" | "relay" | "srflx";
249type RTCIceComponent = "rtcp" | "rtp";
250type RTCIceConnectionState = "checking" | "closed" | "completed" | "connected" | "disconnected" | "failed" | "new";
251type RTCIceCredentialType = "password";
252type RTCIceGathererState = "complete" | "gathering" | "new";
253type RTCIceGatheringState = "complete" | "gathering" | "new";
254type RTCIceProtocol = "tcp" | "udp";
255type RTCIceTcpCandidateType = "active" | "passive" | "so";
256type RTCIceTransportPolicy = "all" | "relay";
257type RTCIceTransportState = "checking" | "closed" | "completed" | "connected" | "disconnected" | "failed" | "new";
258type RTCPeerConnectionState = "closed" | "connected" | "connecting" | "disconnected" | "failed" | "new";
259type RTCPriorityType = "high" | "low" | "medium" | "very-low";
260type RTCRtcpMuxPolicy = "require";
261type RTCRtpTransceiverDirection = "inactive" | "recvonly" | "sendonly" | "sendrecv" | "stopped";
262type RTCSdpType = "answer" | "offer" | "pranswer" | "rollback";
263type RTCSignalingState = "closed" | "have-local-offer" | "have-local-pranswer" | "have-remote-offer" | "have-remote-pranswer" | "stable";
264type RTCStatsIceCandidatePairState = "failed" | "frozen" | "in-progress" | "inprogress" | "succeeded" | "waiting";
265type RTCStatsType = "candidate-pair" | "certificate" | "codec" | "csrc" | "data-channel" | "inbound-rtp" | "local-candidate" | "media-source" | "outbound-rtp" | "peer-connection" | "remote-candidate" | "remote-inbound-rtp" | "remote-outbound-rtp" | "track" | "transport";
266
267
268 createEvent(eventInterface: "RTCDTMFToneChangeEvent"): RTCDTMFToneChangeEvent;
269 createEvent(eventInterface: "RTCDataChannelEvent"): RTCDataChannelEvent;
270 createEvent(eventInterface: "RTCPeerConnectionIceErrorEvent"): RTCPeerConnectionIceErrorEvent;
271 createEvent(eventInterface: "RTCPeerConnectionIceEvent"): RTCPeerConnectionIceEvent;
272 createEvent(eventInterface: "RTCTrackEvent"): RTCTrackEvent;